I Love Optimisation
I love optimisation. So much in fact that I made my own algorithm for finding pi. Its written in rust and at a resolution of 24 can calculate 14 digits of pi in an average of 470 nano seconds (0.00047 milliseconds). Pretty fast right? Well if you turn on compiler optimisation you get an average of 34 nano seconds!!!
But enough of my blabbering. Heres the code:
fn quick_pi(res: i8) -> f64 {
let mut x: f64 = 1.0;
let mut y: f64 = 0.0;
for _ in 1..res {
x *= 0.5;
y = (y + 1.0) * 0.5;
let length = 1.0/((x*x + y*y)).sqrt();
x *= length;
y *= length;
}
(x*x + (y-1.0)*(y-1.0)).sqrt() * (2_i128.pow(res as u32)) as f64
}
How it works is that you get the mid point of 2 points on a circle, normalise it so that points on the circle and repeat for the desired accuracy. This aproximates the curve once we measure the distance between the points.
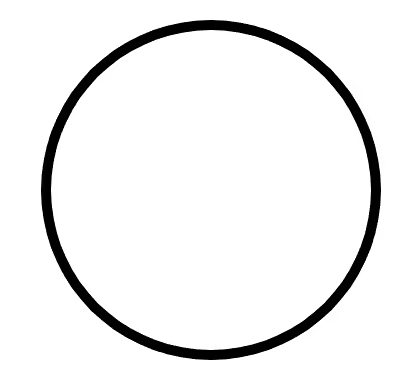
To get pi we have to multiply by the length we cut out. That length being 2^resolution since each time we get the mid point we are dividing the length by 2. And as a result we get pi! Well an aproximation of pi. But at a resolution of 24 we fill up all 14 digits of a f64 (also known as a double) in 34 nano seconds, which I think is cool.